Prerequisites
Before we begin, make sure you have the following:
- Node.js installed
- React Native CLI installed
- A basic understanding of React Native and JavaScript
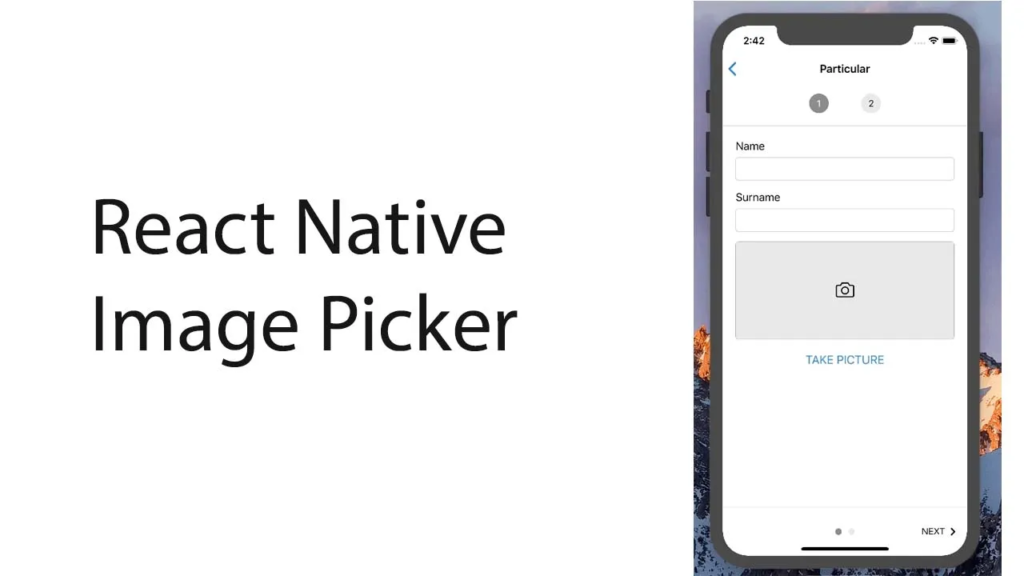
Step 1: Install React Native Image Picker
To install React Native Image Picker, navigate to the root of your project and run the following command:
npm install react-native-image-picker --save
or
yarn add react-native-image-picker
This command will copy all the dependencies into your node_modules
directory.
Step 2: Link React Native Image Picker
To use the React Native Image Picker library, we need to link some dependencies in the Android and iOS project files. Run the following command at the root of the project:
react-native link react-native-image-picker
You will also need to add some permissions on Android.
Step 3: Implement React Native Image Picker
Now that we have installed and linked React Native Image Picker, let’s see how to use it in our application.
Android Permissions
Add the following permissions to your AndroidManifest.xml
file:
<uses-permission android:name="android.permission.CAMERA"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
iOS Permissions
Add the following permissions to your Info.plist
file:
<key>NSCameraUsageDescription</key>
<string>Your message to the user when the camera is accessed.</string>
<key>NSPhotoLibraryUsageDescription</key>
<string>Your message to the user when the photo library is accessed.</string>
<key>NSMicrophoneUsageDescription</key>
<string>Your message to the user when the microphone is accessed.</string>
Implementing the Image Picker
Create a new file called ImagePicker.js
and add the following code:
import React, { Component } from 'react';
import { View, Text, TouchableOpacity } from 'react-native';
import ImagePicker from 'react-native-image-picker';
class ImagePickerExample extends Component {
constructor(props) {
super(props);
this.state = {
imageSource: null,
};
}
selectImage = () => {
const options = {
title: 'Select Image',
storageOptions: {
skipBackup: true,
path: 'images',
},
};
ImagePicker.showImagePicker(options, response => {
if (response.didCancel) {
console.log('User cancelled image picker');
} else if (response.error) {
console.log('ImagePicker Error: ', response.error);
} else if (response.customButton) {
console.log('User tapped custom button: ', response.customButton);
} else {
const source = { uri: response.uri };
this.setState({
imageSource: source,
});
}
});
};
render() {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<TouchableOpacity onPress={this.selectImage}>
<Text>Select Image</Text>
</TouchableOpacity>
{this.state.imageSource && (
<Image
source={this.state.imageSource}
style={{ width: 200, height: 200, marginTop: 20 }}
/>
)}
</View>
);
}
}
export default ImagePickerExample;
In this example, we have created a TouchableOpacity
component that, when pressed, opens the image picker. We then display the selected image using the Image
component.
Conclusion
In this blog, we have covered how to install and use the React Native Image Picker library. We have also provided step-by-step instructions with screenshots and code snippets to help you get started. With this library, you can easily select images from the device’s camera roll or take new photos using the camera.
Happy coding!