What is Fetch?
The Fetch API is a built-in JavaScript interface for making asynchronous HTTP requests to servers. It provides a simple and powerful way to fetch resources from the network, such as JSON data, HTML, or even files.
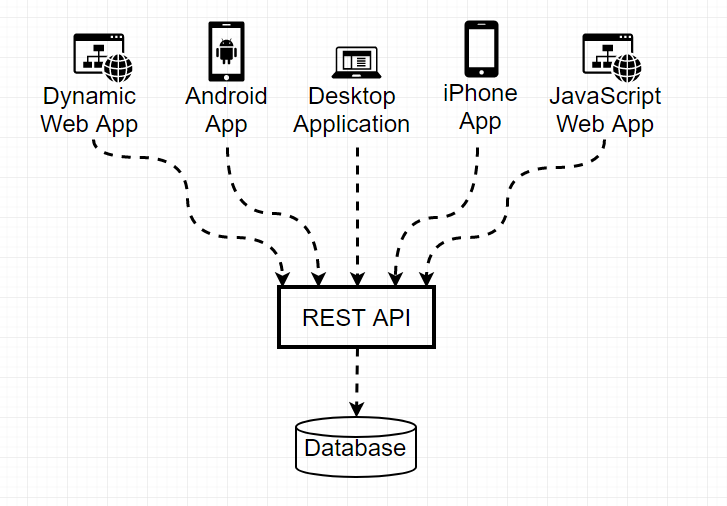
Basic Fetch Request
The most simple use of Fetch is a GET request, which can be done like this:
// Making a GET request using Fetch
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error('There was a problem with the fetch operation:', error);
});
In this example, we’re making a GET request to ‘https://api.example.com/data‘. The fetch()
function returns a Promise that resolves to the Response object representing the response to the request.
We use .then()
to handle the response asynchronously. Inside the first .then()
block, we check if the response was successful (status code 200-299) using response.ok
. If it’s not, we throw an error.
If the response is successful, we use the json()
method to parse the response body as JSON. This method also returns a Promise, so we use another .then()
block to access the parsed JSON data.
Handling Errors:
In the above example, we’re using .catch()
to handle any errors that occur during the fetch operation. This is important for gracefully handling network errors or other issues that may arise.
Handling Responses
Fetch returns a Promise that resolves to the Response of a request, whether it is successful or not.
fetch('https://api.example.com/data') .then((response) => console.log(response
Checking Successful Fetch
Often, you will want to check if the request was successful. Here’s how to do it:
fetch('https://api.example.com/data')
.then((response) => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
});
Handling JSON Data
To extract the JSON body content from the response, we use the json() method:
fetch('https://api.example.com/data') .then((response) => response.json()) .then((data) => console.log(data));
Error Handling
If a network error occurs, the catch() is triggered.
fetch('https://api.example.com/data')
.then((response) => response.json())
then ((data) => console.log(data))
.catch((error) =>
console.error('There has been a problem with your fetch operation:', error),
);
Fetch POST Request
Fetch is not just for GET requests. You can use it to send other types of requests, like POST.
fetch('https://api.example.com/data', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ id: '200' }),
});
Conclusion:
The Fetch API is a powerful tool for making HTTP requests in JavaScript. Its simple, promise-based interface makes it easy to fetch data from servers and handle responses asynchronously. By mastering the Fetch API, you can build more responsive and dynamic web applications.