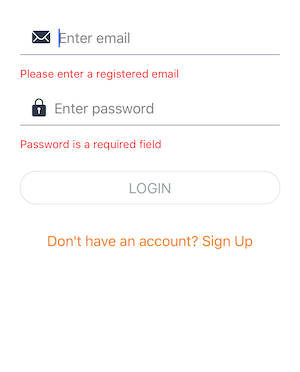
Step 1: Create new Project
First, make sure you have Node.js installed on your system. Then, create a new React app using Create React App:
npx create-react-app my-blog
cd my-blog
Step 2: Install Formik and Yup for form validation:
npm install formik yup
Step 3:
Now, let’s create a login form component using Formik for form handling and Yup for validation.
Create a new file named LoginForm.js
in the src
directory:
// LoginForm.js
import React from 'react';
import { Formik, Form, Field, ErrorMessage } from 'formik';
import * as Yup from 'yup';
const LoginForm = ({ onSubmit }) => {
return (
<Formik
initialValues={{ email: '', password: '' }}
validationSchema={Yup.object().shape({
email: Yup.string().email('Invalid email').required('Email is required'),
password: Yup.string().required('Password is required')
})}
onSubmit={(values, { setSubmitting }) => {
onSubmit(values);
setSubmitting(false);
}}
>
{({ isSubmitting }) => (
<Form>
<div>
<label htmlFor="email">Email</label>
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" />
</div>
<div>
<label htmlFor="password">Password</label>
<Field type="password" name="password" />
<ErrorMessage name="password" component="div" />
</div>
<button type="submit" disabled={isSubmitting}>
Submit
</button>
</Form>
)}
</Formik>
);
};
export default LoginForm;
Step 4:
Now, let’s create a simple login page component that uses this login form.
Create a new file named LoginPage.js
in the src
directory:
// LoginPage.js
import React from 'react';
import LoginForm from './LoginForm';
const LoginPage = () => {
const handleSubmit = (values) => {
console.log(values); // You can handle login logic here
};
return (
<div>
<h2>Login</h2>
<LoginForm onSubmit={handleSubmit} />
</div>
);
};
export default LoginPage;
Step 5:
Finally, let’s modify App.js
to include the LoginPage
component:
// App.js
import React from 'react';
import './App.css';
import LoginPage from './LoginPage';
function App() {
return (
<div className="App">
<LoginPage />
</div>
);
}
export default App;
Now, you have a basic login page with form validation using Formik in your React.js application. You can expand upon this by adding more features like registration, authentication, and integrating it with a backend server for real-world usage.
It will part if we began to lot of